In this module we introduce the notions of abstraction and accumulators. First we show how recursive functions can be rewritten to maintain information during each recursive call using accumulators. Second we upgrade to the Intermediate Student Language which allows functions as values. We then show how functions can consume/produce other functions allowing for more abstract, and more elegant, designs.
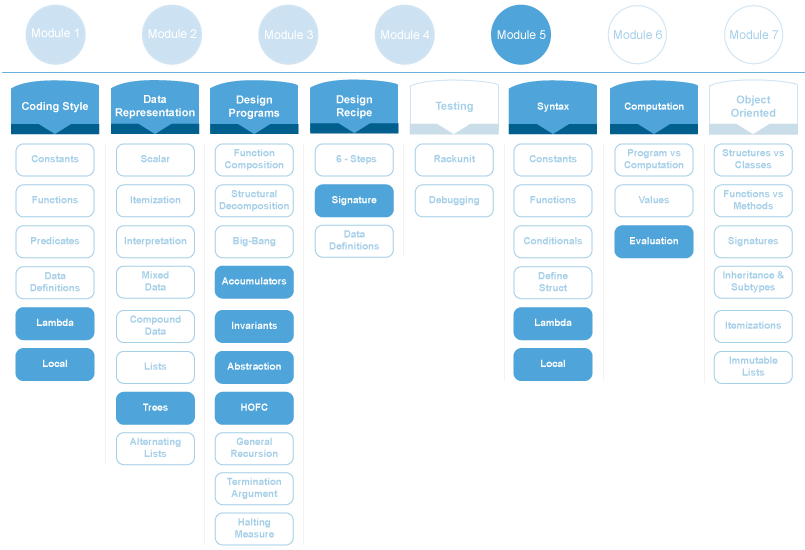
- Given a function that uses mutual recursion, convert it to use accumulators.
- Document accumulator arguments by writing an invariant.
- Write a function definition that is local in scope to another function.
- Given a set of Scheme functions that contain duplicative code, write the abstracted function.
- Given a data definition for tree structured data, write a template.
- Given a specification for information represented as a tree, write a data definition that maps the information into Scheme data.
- Recognize patterns for built-in abstract functions.
- Write the contracts for the built-in abstract functions.
- Given abstract functions, use Higher Order Function Composition to define a new function.
- Given a function that uses structural decomposition, convert it into a function that uses Higher Order Function Composition.
- Given a signature that takes other functions as arguments, write down valid inputs for the signature.
- Given a recursive data definition, write a fold function.
- Recognize patterns for built-in abstract functions.
- Write the contracts for the built-in abstract functions.
- Given abstract functions, use Higher Order Function Composition to define a new function.
- Given a signature that takes other functions as arguments, write down valid inputs for the signature.
- Given a function that uses structural decomposition, convert it into a function that uses Higher Order Function Composition.
- Given a recursive data definition, write a fold function.
- Write an anonymous function using the keyword lambda.
- Write a call to an anonymous function with the appropriate number of inputs.
- Given a Scheme expression, write down the sequence of steps taken during evaluation.
- Use the syntactical conventions for function definitions.
- Given a Scheme function, identify the strategy used.
- Development through Iterative Refinement
- Processing Two Complex Pieces of Data
- Intermezzo 3: Local Definitions and Lexical Scope
- Similarities in Definitions
- Functions are Values
- Designing Abstractions from Examples
- Designing Abstractions from First-Class Functions
- The Loss of Knowledge
- Designing Accumulator-Style Functions
- More Uses of Accumulation
- DUE DATE: Monday November 20th at 11:59pm
S-Expressions
You are given the following data definition
;; An S-Expression (SExp) is one of
;; - Atom
;; - List<SExp>
;; INTERP: represents an s-expression
;; An Atom is one of
;; - Number
;; - String
;; - Symbol
;; - Boolean
;; INTERP: represents atomic values
-
Provide the deconstructor template for
SExp
-
Design a function that given an
SExp
returns the total number ofAtom
s in theSExp
. -
Design a function that given an
SExp
returns a list of all Symbols in theSExp
. -
Design a function that given an
SExp
returns the sum of all Numbers in theSExp
. -
Design a function that given an
SExp
and two stringss1
ands2
returns a newSExp
where all instance ofs1
have been replaced withs2
.
Higher Order Functions
DrRacket has lots of abstract functions for processing lists (pg. 313, Sect. 21.2, or the online version).
Given the following data definitions:
(define-struct grade (letter num))
;; A Grade is: (make-grade LetterGrade Number)
;; INTERP: represents a homework grade as
;; a letter grade and number grade
;; A LetterGrade is one of:
;; - 'A >= 90
;; - 'B >= 80
;; - 'C >= 70
;; - 'D >= 60
;; - 'F < 60
;; A List<Grades>
(define grades (list (make-grade 'D 62) (make-grade 'C 79)
(make-grade 'A 93) (make-grade 'B 84)
(make-grade 'F 57) (make-grade 'F 38)
(make-grade 'A 90) (make-grade 'A 95)
(make-grade 'C 76) (make-grade 'A 90)
(make-grade 'F 55) (make-grade 'C 74)
(make-grade 'A 92) (make-grade 'B 86)
(make-grade 'F 43) (make-grade 'C 73)))
Design the requested functions to manipulate Grades
. You must use the
given list as one of your tests.
For each you may use a local expressions.
Note: if you do not use the DrRacket higher order functions from Section 21.2 in the book, you will not receive credit for the sub-problem!
-
Design the function
log->lon
that converts aList<Grade>
into aList<Number>
that contains just the numerical grade, using the Scheme functionmap
. -
Using
foldr
, design the functionbest-grade
that finds the highest Grade in aList<Grade>
. -
Design a function
just-As
that returns a list of only the 'A grades, usingfilter
. -
Use
andmap
to design the functionall-pass?
that checks to see if all the Grades in a given list are not'F
. -
Finally design the function
bonus
that adds 5 to all of the Grades in a given list, and updates the letter portion of the Grade if it changes. Usemap
to design your function... it must return aList<Grade>
!
Trees
A friend of your found this online quiz that they are trying to solve and they are stuck. Help them out by solving these questions.
Provide your solutions without using any abstract functions.
-
Provide a data definition for a Binary Search Tree that contains non-negative Integers (BSTI) and answer the following
questions
- Design a function that given a BSTI returns the sum of all the integers found inside the BSTI.
- Design a function that given a BSTI returns the product of all the integers found inside the BSTI.
-
Design a funciton that given a BSTI and a non negative integer
dx
multiplies each integer in the BSTI bydx
.
-
Provide a data definition for a Binary Search Tree that contains Strings (BSTS). Your BSTS should
use alphabetical ordering to decide which string should be stored to the left or right subtree.
Answer the following questions for a BSTS
- Design a function that given a BSTS appends all the strings in the BSTS using an in-order traversal.
-
Design a function that given a BSTS and a String
ds
appendsds
to each string in the BSTS.
-
Provide a data defintion for a tuple. A tuple always contains 2 values, the first value is a string and the second
value is a non-negative integer. Provide a data definition of a Binary Search Tree that can contain Tuples (BSTT).
Your BSTT should use the alphabetical ordering on the String inside the Tuple's in order to decide which tuple
should be stored to the left or right subtree of a node.
Answer the following questions on BSTT
- Design a function that given a BSTT returns the sum of all Integer values in the Tuples found in the BSTT.
- Design a function that given a BSTT appends all the String values in the Tuples found int he BSTT using an in-order traversal.
- Design a function that given a BSTT returns a BSTS that holds only the String values found in each Tuple in the BSTT.
Now that you have a solution for the 3 different Binary Search Trees we would like to generalize (or abstract) over the data definition and your solution(s).
- Provide a generic data definition for a BST (GBST) that can take any kind of data as an element.
- The solutions to 1.c., 2.b., and 3.c. are similar. Use your existing solutions to design a generic function on GBST. Use your generic function to provide a second solution to 1.c., 2.b., 3.c.
- The solutions to 1.a., 1.b., 2.a., 3.a. and 3.b are similar. Use your existing solutions to design a generic function on GBST. Use your generic function to provide a second solution to 1.a., 1.b., 2.a., 3.a. and 3.b.