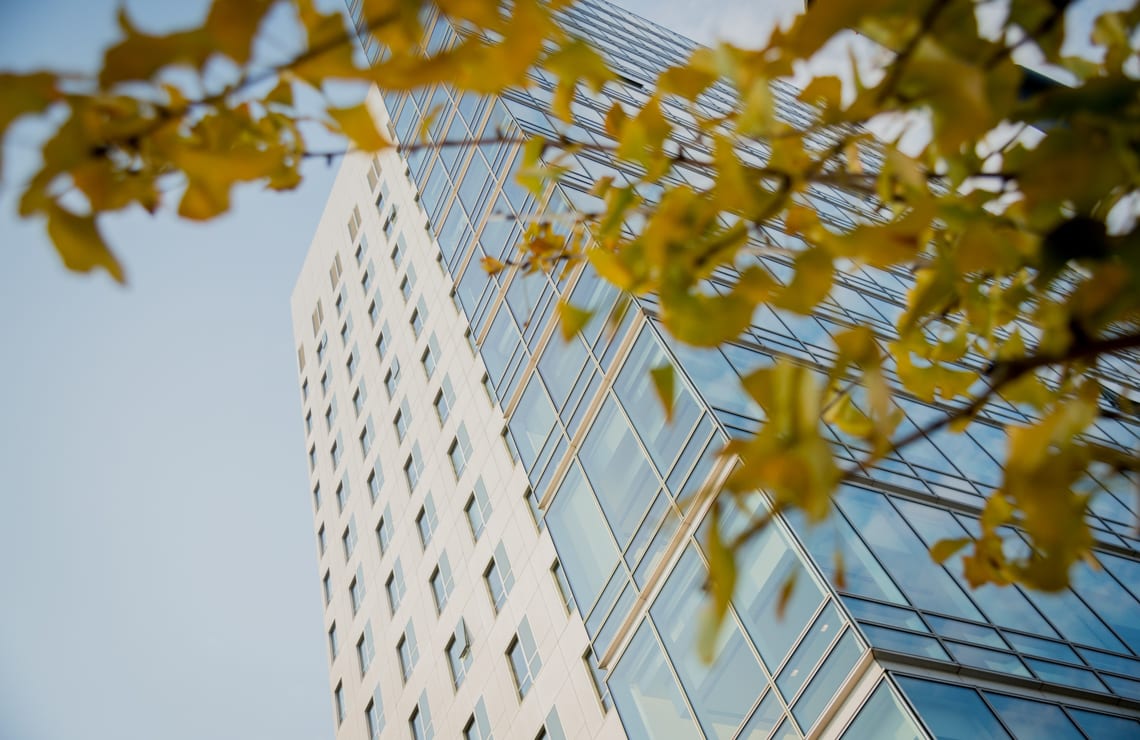
CS5002: Discrete Structures Course Charter
Introduction
CS5002 is a course meant to provide Align students with an introduction to discrete mathematics, as well as some data structures and algorithms. This document is meant to provide guidance for future instructors of CS5002, laying out how the goals of the course fit in with the rest of the Align program, the learning objectives currently considered most important, and additional thoughts on how the class can be taught effectively.
Goals
CS5002 is meant to be taken alongside the Align students’ first introduction to software development (i.e., CS5001). Its primary purpose is to introduce students to discrete mathematics. Its secondary purpose it to touch on data structures and algorithms in preparation for CS5004 and CS5006, which further introduce both topics.
Since this course is typically taken alongside CS5001, faculty teaching 5002 can assume some knowledge of Python, particularly in the latter half of the semester. Part-time students should be advised to take 5001 before 5002.
High-Level Learning Objectives
At the end of 5002, a student should be able to do the following:
- Convert between decimal, binary, and hex.
- Understand two’s complement representations of negative numbers.
- Read and write mathematical logical formulas.
- Use logical operators effectively.
- Understand the set operators of union, intersection, and complement.
- Understand relations and functions.
- Perform basic counting tasks – e.g. counting possible passwords – using permutations and combinations as appropriate.
- Calculate basic probabilities by counting success outcomes and total outcomes.
- Apply proof techniques, particularly mathematical induction.
- Model problems using trees and graphs.
- Describe the number of operations in an algorithm as a function of the input size, and identify superpolynomial running times as intractable.
- Understand the meaning of big-O notation.
Implementation Details
The course evaluates students through
- Weekly problem sets.
- Exams. There are typically 2 exams: midterm and final.
Because students are typically enrolled in CS5001 in parallel with this course, faculty may assume some knowledge of Python, particularly in the latter part of the semester, and may therefore include programming problems in weekly assignments. Indeed, faculty teaching CS5002 are encouraged to include some implementation in the latter part of the course.
Topics and Learning Outcomes
Note that the topics and learning outcomes are the minimum to be achieved in CS5002. Faculty may choose to present additional material or cover a topic in greater depth. The order of topics here does not imply an ordering for the course itself. Faculty are encouraged to develop syllabi that complement their teaching style. Faculty are also encouraged to share syllabi and other materials with each other.
A note on levels of mastery, which are taken from CS2013 (see cs2013.org): For levels of mastery, CS2013 takes inspiration from (but does not directly follow) Bloom’s Taxonomy. CS2013 defines three levels:
- Familiarity: The student understands what a concept is or what it means. This level of mastery concerns a basic awareness of a concept as opposed to expecting real facility with its application. It provides an answer to the question “What do you know about this?”
- Usage: The student is able to use or apply a concept in a concrete way. Using a concept may include, for example, appropriately using a specific concept in a program, using a particular proof technique, or performing a particular analysis. It provides an answer to the question “What do you know how to do?”
- Assessment: The student is able to consider a concept from multiple viewpoints and/or justify the selection of a particular approach to solve a problem. This level of mastery implies more than using a concept; it involves the ability to select an appropriate approach from understood alternatives. It provides an answer to the question “Why would you do that?”
Note that course topics appear in regular font. Learning outcomes appear in italics.
Also note that some learning outcomes apply to many course topics. More specific learning outcomes appear with their respective topics.
Structural inductionState the relationship between ideas of mathematical and/or structural induction to recursion and recursively defined structures. [Familiarity]
Course Topics | Learning Outcomes |
---|---|
Binary/Hexadecimal representation of numbers
|
|
Basic Logic
|
|
Sets
Relations
Functions
|
|
Counting arguments
Solving recurrence relations |
|
Permutations and combinations |
|
The pigeonhole principle | Apply the pigeonhole principle in the context of a formal proof. [Usage] |
Discrete Probability
|
|
Basic modular arithmetic | Perform computations involving modular arithmetic. [Usage] |
Notion of contrapositive
|
|
Trees and Graphs (tie with Structural Induction) |
|
Basic Analysis
Big O notation
|
|