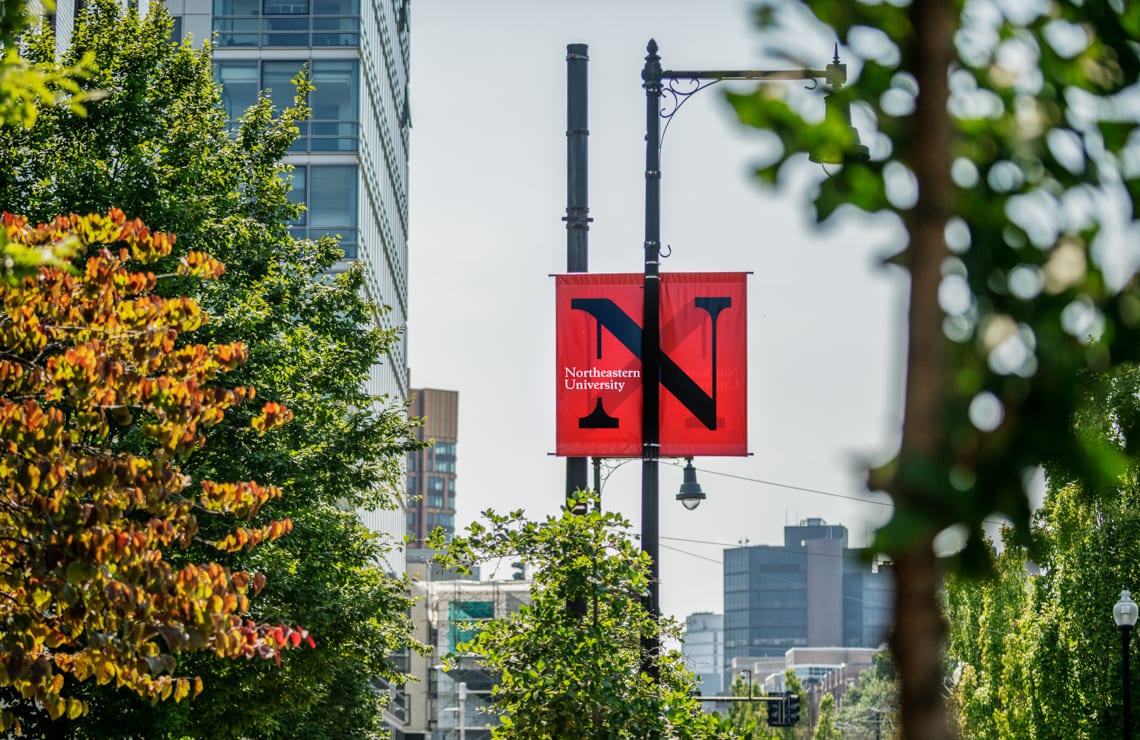
CS5001: Intensive Foundations of CS Course Charter
Introduction
CS5001 is an introduction to computing and programming. The course is meant to introduce the basic rules of computation and the principles of systematic problem solving through programming.
The aim of this document is to lay out the objectives and outcomes for CS5001.
Goals
The course serves as the first programming course in the Align program that focuses on program design. Align students vary in their computing background. The course aims to provide a more uniform computing background for each student by introducing the student to programming and program design and/or by augmenting (and sometimes correcting) students’ pre-existing knowledge in the field.
Specifically:
Experience with problem solving through programming. Get comfortable with reading, understanding and analyzing fairly detailed problem statements. Decompose and propose a solution. Code the proposed solution. Test, debug, iterate.
Experience with elementary data structures and algorithmic techniques. Gain exposure to and practice with elementary data structures as well as algorithmic techniques. Begin to understand the consequences (time and space implications) of different algorithms and implementations.
High-Level Learning Objectives
The course’s outcomes are captured in the following list of high-level learning objectives.
- Write correct and clearly documented small- to medium-sized programs that others can read, understand, and modify.
- Read one’s own and others’ code; trace execution; determine functionality of short segments of code.
- Break large problems into an appropriate design for implementation.
- Use generalization for data and functions to limit code duplication.
- Select appropriate data types to represent information.
- Develop tests to exercise implemented code and appreciate the importance of good testing in the software development process.
- Appreciate the impact of data structure and algorithm choice on the running time and storage space needed to run a program.
- Be familiar with common library classes; use objects and methods.
Implementation Details
The programming language used in this course is Python. Languages used in other Align bridge courses include Java (in CS5004: Object Oriented Design) and C (in CS5007: Systems, as well as CS5006: Algorithms). The choice of Python in 5001 is due to its accepted utility in industry, as well as its C-like syntax, which should allow students to more easily move among the languages used in the bridge.
The course evaluates students through
- Weekly programming assignments. Toward the end of the term, it is helpful to run a multi-week assignment (or a large assignment with weekly deliverables) that gives students the experience of working on a larger project.
- Weekly lab sessions.
- Exams. There are typically 2 exams, midterm and final.
Topics and Learning Outcomes
Note that the topics and learning outcomes are the minimum to be achieved in CS5001. Faculty may choose to present additional material or cover a topic in greater depth. The order of topics here does not imply an ordering for the course itself. Faculty are encouraged to develop syllabi that complement their teaching style. Faculty are also encouraged to share syllabi and other materials with each other.
A note on levels of mastery, which are taken from CS2013 (see cs2013.org): For levels of mastery, CS2013 takes inspiration from (but does not directly follow) Bloom’s Taxonomy. CS2013 defines three levels:
- Familiarity: The student understands what a concept is or what it means. This level of mastery concerns a basic awareness of a concept as opposed to expecting real facility with its application. It provides an answer to the question “What do you know about this?”
- Usage: The student is able to use or apply a concept in a concrete way. Using a concept may include, for example, appropriately using a specific concept in a program, using a particular proof technique, or performing a particular analysis. It provides an answer to the question “What do you know how to do?”
- Assessment: The student is able to consider a concept from multiple viewpoints and/or justify the selection of a particular approach to solve a problem. This level of mastery implies more than using a concept; it involves the ability to select an appropriate approach from understood alternatives. It provides an answer to the question “Why would you do that?”
Note that course topics appear in regular font. Learning outcomes appear in italics.
Also note that some learning outcomes apply to many course topics. More specific learning outcomes appear with their respective topics.
Course Topics | Learning Outcomes |
---|---|
Basic syntax and semantics of a higher-level language
|
|
Variables and primitive data types (e.g., numbers, characters, Booleans) |
|
File I/O |
|
Conditional and iterative control structures |
|
Strings, Lists, Tuples, Sets, Dictionaries
|
|
Recursion |
|
Program correctness
|
|
Objects and methods
|
|
Sequential and binary search algorithms
|
|
Stacks and Queues (use natural list structure of Python) |
|